Httpsession Serialization
- Posted in:Admin
- 14/04/18
- 45
Here is an example of what I am doing except this example works when the session state mode is set to InProc, but when changed to StateServer it does not.
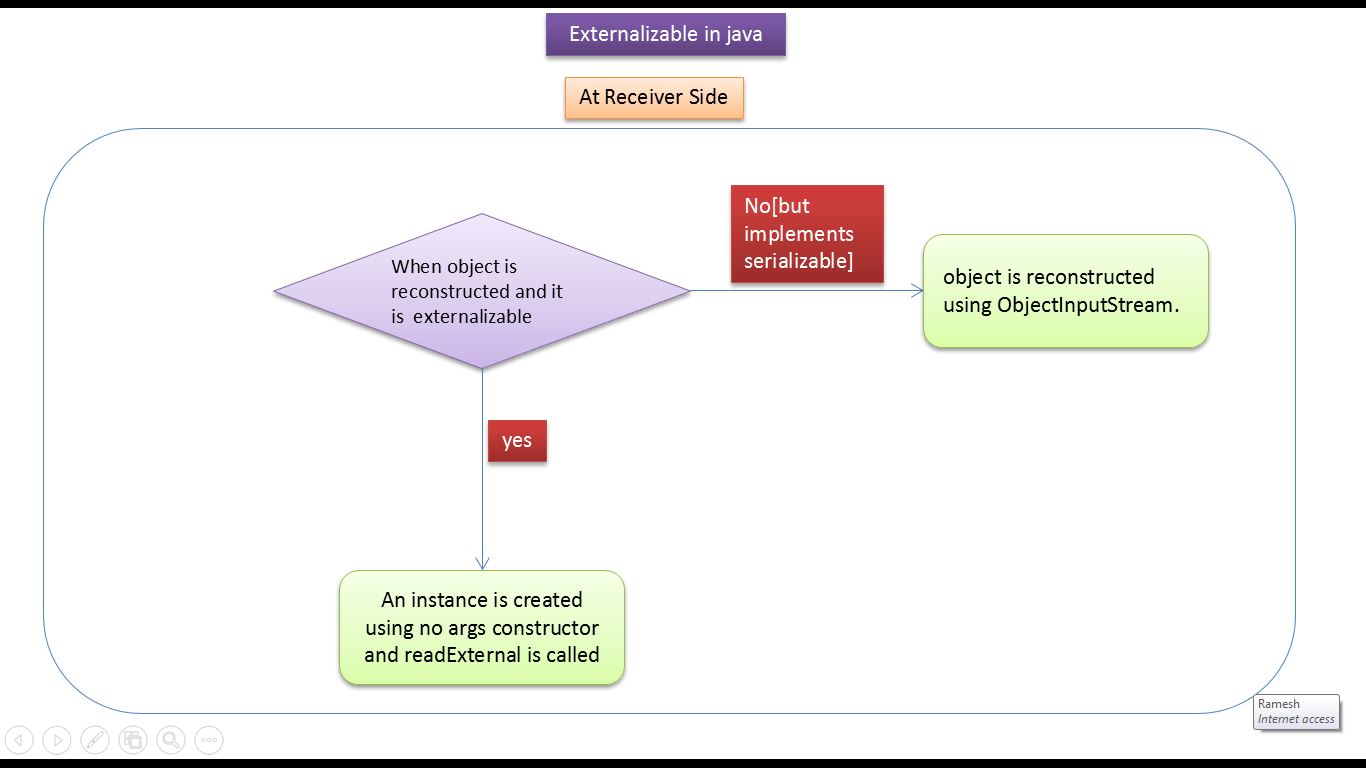
Warning You should only use cache-based sessions if you’re using the Memcached cache backend. The local-memory cache backend doesn’t retain data long enough to be a good choice, and it’ll be faster to use file or database sessions directly instead of sending everything through the file or database cache backends. Additionally, the local-memory cache backend is NOT multi-process safe, therefore probably not a good choice for production environments. If you have multiple caches defined in, Django will use the default cache. To use another cache, set to the name of that cache. Once your cache is configured, you’ve got two choices for how to store data in the cache: • Set to 'django.contrib.sessions.backends.cache' for a simple caching session store.
Session data will be stored directly in your cache. However, session data may not be persistent: cached data can be evicted if the cache fills up or if the cache server is restarted. • For persistent, cached data, set to 'django.contrib.sessions.backends.cached_db'. This uses a write-through cache – every write to the cache will also be written to the database. Session reads only use the database if the data is not already in the cache. Both session stores are quite fast, but the simple cache is faster because it disregards persistence.
In most cases, the cached_db backend will be fast enough, but if you need that last bit of performance, and are willing to let session data be expunged from time to time, the cache backend is for you. If you use the cached_db session backend, you also need to follow the configuration instructions for the.
Warning If the SECRET_KEY is not kept secret and you are using the, this can lead to arbitrary remote code execution. An attacker in possession of the can not only generate falsified session data, which your site will trust, but also remotely execute arbitrary code, as the data is serialized using pickle. If you use cookie-based sessions, pay extra care that your secret key is always kept completely secret, for any system which might be remotely accessible. The session data is signed but not encrypted When using the cookies backend the session data can be read by the client.
A MAC (Message Authentication Code) is used to protect the data against changes by the client, so that the session data will be invalidated when being tampered with. The same invalidation happens if the client storing the cookie (e.g. Your user’s browser) can’t store all of the session cookie and drops data. Even though Django compresses the data, it’s still entirely possible to exceed the per cookie. No freshness guarantee Note also that while the MAC can guarantee the authenticity of the data (that it was generated by your site, and not someone else), and the integrity of the data (that it is all there and correct), it cannot guarantee freshness i.e. That you are being sent back the last thing you sent to the client.
Stardock Fences Portable Tv. This means that for some uses of session data, the cookie backend might open you up to. Unlike other session backends which keep a server-side record of each session and invalidate it when a user logs out, cookie-based sessions are not invalidated when a user logs out. Thus if an attacker steals a user’s cookie, they can use that cookie to login as that user even if the user logs out. Cookies will only be detected as ‘stale’ if they are older than your. Performance Finally, the size of a cookie can have an impact on the. Using sessions in views When SessionMiddleware is activated, each object – the first argument to any Django view function – will have a session attribute, which is a dictionary-like object.
You can read it and write to request.session at any point in your view. You can edit it multiple times. Class backends.base. SessionBase This is the base class for all session objects. It has the following standard dictionary methods: __getitem__( key) Example: fav_color = request.session['fav_color'] __setitem__( key, value) Example: request.session['fav_color'] = 'blue' __delitem__( key) Example: del request.session['fav_color']. This raises KeyError if the given key isn’t already in the session.
__contains__( key) Example: 'fav_color' in request.session get( key, default=None) Example: fav_color = request.session.get('fav_color', 'red') pop( key, default=__not_given) Example: fav_color = request.session.pop('fav_color', 'blue') keys() items() setdefault() clear() It also has these methods: flush() Deletes the current session data from the session and deletes the session cookie. This is used if you want to ensure that the previous session data can’t be accessed again from the user’s browser (for example, the function calls it). Set_test_cookie() Sets a test cookie to determine whether the user’s browser supports cookies.